Force Android in App Updates
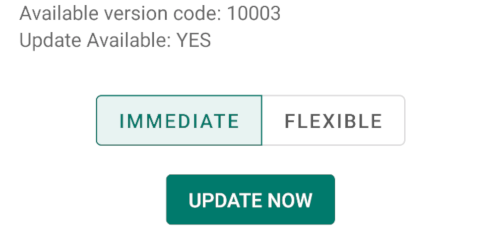
With the new Play Core Library Google offers us the possibility to prompt for app updates from within our app for the first time. Users get informed about new available versions and can decide to update their app. If needed, we can even force the update altogether. The Google library itself handles the download and installation of the new app version.
There are two modes how an update can be triggered:
- Flexible: The app update runs in the background. The User can continue using the app and the update will be applied when the user chooses to restart the app.
- Immediate: The user is forced to update the app and the app is unusable until the process is finished.
The downside is, that the library itself is not able to detect if an update should be flexible or immediate. Sometimes it might be necessary to force users to update right away due to some breaking changes or unwanted crashes.
So we need to provide a way to decide between a flexible or immediate update. To simplify this process we published an Android library which allows us to decide on the type of update we need.
Who decides to force an update?
The library offers an easy way to detect if an update should be forced. All we have to do is implement ForceUpdateProvider
and call doUpdate()
when we need to update right away.
class DemoForceUpdateProvider : ForceUpdateProvider {
override fun requestUpdateShouldBeImmediate(availableVersionCode: Int, doUpdate: () -> Unit) {
// place your logic here
// obtain force update decision from your source eg Firebase Remote Config
// if a forced update is needed, just call doUpdate
doUpdate()
}
}
inAppUpdateManager = InAppUpdateManager(this, DemoForceUpdateProvider())
There are a lot of possible sources to decide if an update should be forced. Examples are Firebase Remote Config
, File hosted on your server
, Even/Odd available version code
, …
Since the forced-update screen that shows when we use this method can be closed through the back button, we need to override onActivityResult
to keep showing the update again to achieve an actually blocking update mechanism.
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
inAppUpdateManager.onActivityResult(requestCode, resultCode)
super.onActivityResult(requestCode, resultCode, data)
}
That’s it. The end result looks like this:
The library is available on GitHub: https://github.com/allaboutapps/InAppUpdater